TL-B 语言
TL-B(类型语言 - 二进制)用于描述类型系统、构造函数和现有功能。例如,我们可以使用 TL-B 方案构建与 TON 区块链关联的二进制结构。特殊的 TL-B 解析器可以读取方案,将二进制数据反序列化为不同的对象。TL-B 描述了 Cell
对象的数据方案。如果您不熟悉 Cells
,请阅读 Cell & Bag of Cells(BOC) 文章。
概述
我们将任何一组 TL-B 构造称为 TL-B 文档。一个 TL-B 文档通常包括类型的声明(即它们的构造函数)和功能组合子。每个组合子的声明都以分号 (;
) 结尾。
这是一个可能的组合子声明示例:
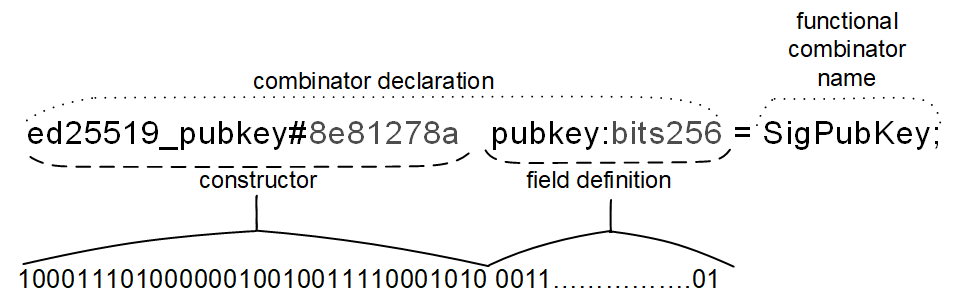
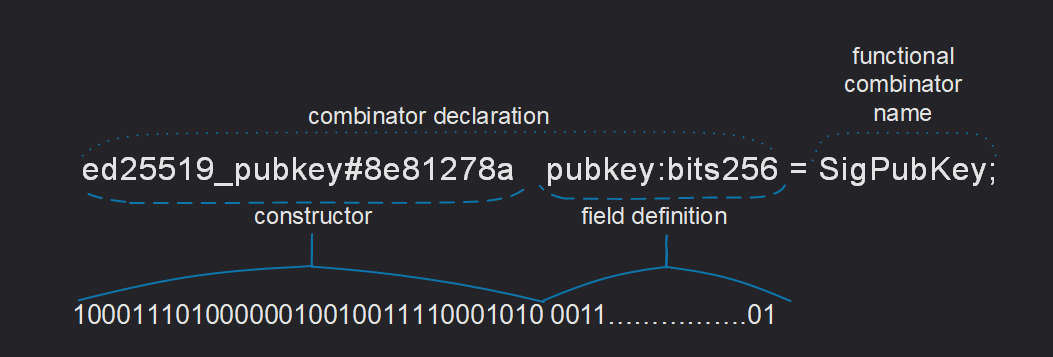