开始使用 TON
从零开始在 TON 区块链上建立您的第一个应用程序,了解其速度、可靠性和异步思维的基本概念。
如果您是编程新手,本指南将是您的最佳选择。
本学习路径包含 5 个模块,大约需要 45 分钟。
🛳 你将学到什么
在本教程中,您将学习如何使用 JavaScript 轻松进行区块链交易。虽然不通过本教程也能学会,但这种方法既方便又友 好。
- 您将使用 Tonkeeper 制作自己的 TON 钱包
- 您将使用 Testnet faucet为钱包充值,以便进行测试。
- 您将了解 TON 智能合约的基本概念 (Addresses, Cells)
- 您将学习如何使用 TypeScript SDK 和 API 提供商与 TON 交互
- 您将使用 NFT Miner 控制台应用程序编译第一笔交易
你要去开采一个 NFT 火箭成就!!
作为 TON 的第一批矿工,你们将通过工作证明智能合约,最终为你们的 TON 钱包挖出秘密奖励。看看吧
我们今天的目标是挖掘一个 NFT!这个成就将与您 永远 同在。
最后,即使在主网中,您也能挖掘到这一 NFT 成就。(成本仅为 0.05 TON !)。
在 TON 区块链上挖矿
今天,我们将教我们的潜在建设者如何在 TON 区块链上挖矿。这次体验将让大家了解挖矿的意义,以及为什么比特币挖矿有助于彻底改变这个行业。
PoW Giver 智能合约框架定义了最初的挖矿流程,为 TON 奠定了基础,虽然该框架在发布时已经完成,但最后一个 TON 于 2022 年 6 月挖出,结束了 TON 的工作证明(PoW)代币分配机制。尽管如此,随着我们最近过渡到权益证明(PoS),TON 的权益证明时代才刚刚开始。
现在,让我们专注于成为TON 开发者的第一步,学习如何在 TON 上挖掘 NFT!以下是我们的目标示例。
如果我们专注于手头的工作,大约半小时就能创建一个矿工。
🦄 入门
要开始工作,所有开发人员都将使用以下组件:
- 钱包:在 Testnet 模式下,您需要一个非托管钱包来存储 NFT。
- 仓库:我们将使用专门为您设计的现成模板。
- 开发环境: 开发人员需要确定是使用本地环境还是云环境进行挖矿。
下载并创建钱包
首先,您需要一个可以接收和存储 TON 的非托管钱包。在本指南中,我们使用 Tonkeeper。您需要在钱包中启用Testnet模式,以便接收Testnet通证。这些代币稍后将用于向智能合约发送最后的铸币交易。
对于非托管钱包,用户自己拥有钱包和私钥。
要下载和创建 TON 钱包,请按以下简单步骤操作:
简单!现在我们开始开发。
项目设置
为了简化您的工作,跳过日常的低级任务,我们将使用一个模板。
请注意,您需要 登录 到 GitHub 才能继续工作。
请使用 ton-on-boarding-challenge 模板创建您的项目,方法是点击 “Use this template” 按钮,然后选择 “Create a new repository” 选项卡,如下图所示:
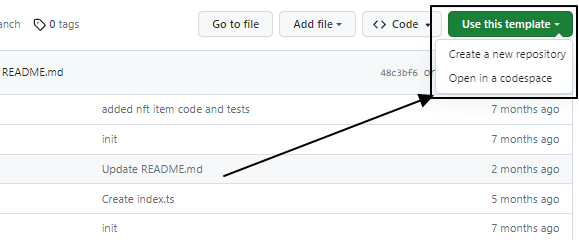

完成此步骤后,您将获得一个高性能的仓库,作为您的矿工核心。祝贺您!✨
开发环境
下一步是选择最适合您的需求、经验水平和整体技能的开发人员环境。正如您所看到的,使用云环境或本地环境都可以完成这一过程。云开发通常被认为更简单、更容易上手。下面,我们将概述这两种方法所需的步骤。
确保已在 GitHub 配置文件中打开了上一步中根据模板生成的仓库。
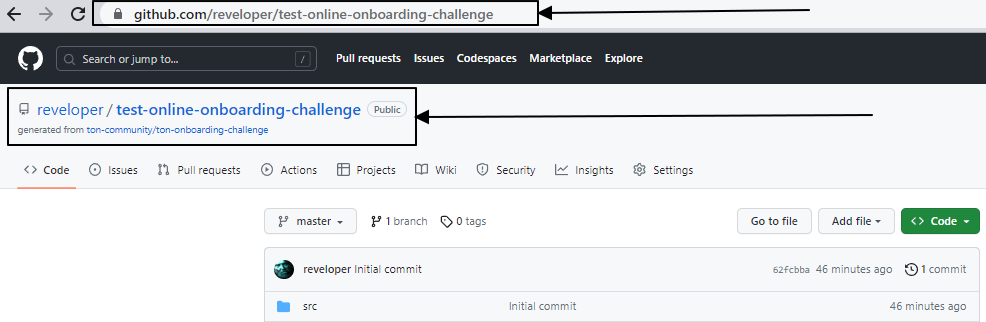
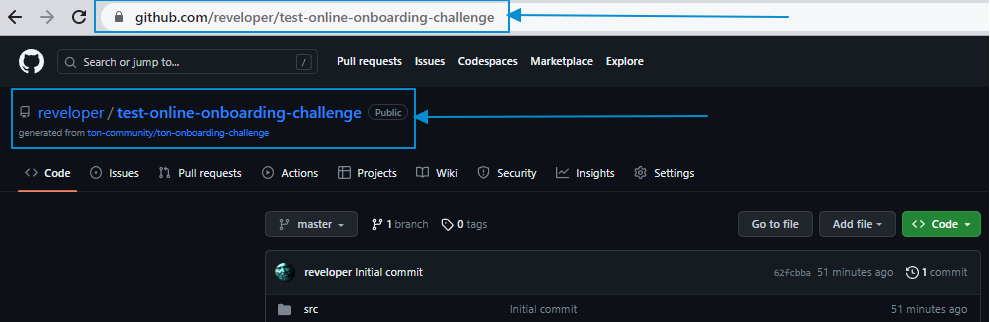
本地和云开发环境
-
对于不熟悉 JavaScript 的用户来说,使用 JavaScript 集成开发环境可能具有挑战性,特别是如果您的计算机和工具系统不是为此目的而配置的。
-
不过,如果你熟悉 NodeJS 和 Git,知道如何使用
npm
,你可能会发现使用 本地环境 会更方便。
云代码空间
如果选择云开发环境,首先选择 Code 选项卡,然后点击 GitHub 仓库中的 Create codespace on master 按钮,就可以轻松开始了,如下图所示:
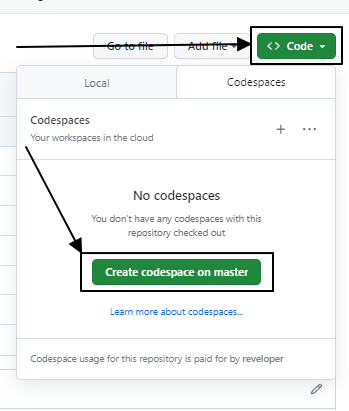
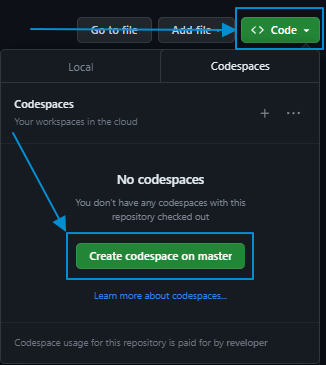
完成这一步后,GitHub 将创建一个特殊的云工作区,允许你访问 VSCode Online IDE(Visual Studio Code 在线集成开发环境)。
一旦访问权限被授予(代码空间通常在 30 秒内启动),您就拥有了开始工作所需的一切,而无需安装 Git、Node.js 或其他开发工具。
本地开发环境
要建立本地开发环境,您需要访问这三个基本工具:
- Git:Git 是每个开发人员处理软 件源时必不可少的工具。可在 此处 下载。
- NodeJS:Node.js 是 JavaScript 和 TypeScript 运行时环境,通常用于在 TON 上进行应用程序开发。可在 此处 下载。
- JavaScript集成开发环境。JavaScript IDE 通常用于在本地开发环境中进行开发。Visual Studio Code(VSCode)就是一个例子。
要开始使用,您需要克隆 GitHub 仓库模板,并在集成开发环境 (IDE) 中打开正确的仓库。
运行脚本
在本指南中,您需要运行 TypeScript 脚本。运行脚本或安装模块等所有命令都通过命令行执行,命令行位于集成开发环境的终端工作区中。该工作区通常位于集成开发环境的底部。
例如,在云代码空间中,应打开终端工作区(如果尚未打开):
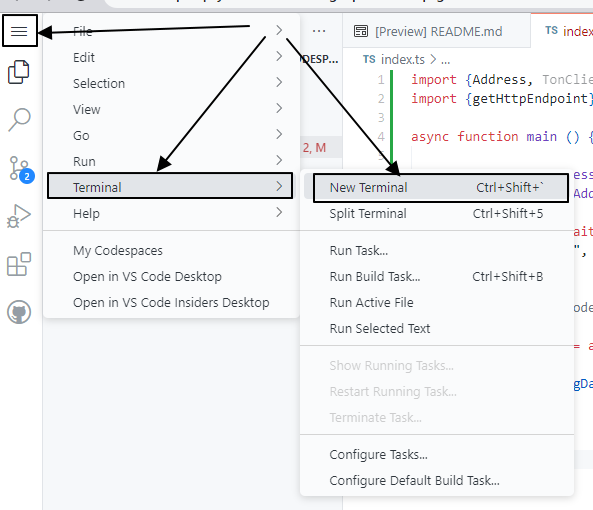
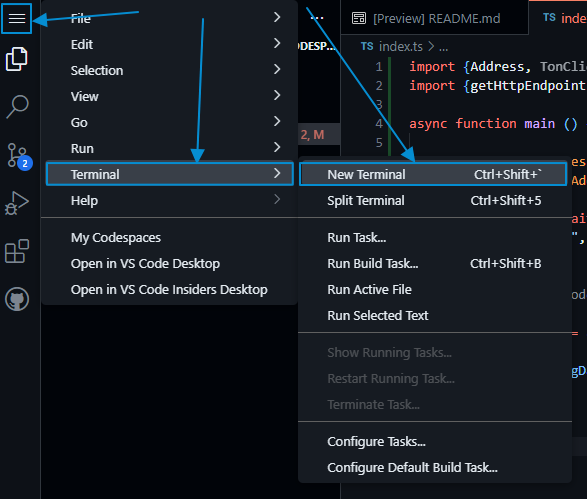
在该窗口中输入命令,并用 Enter 键执行:
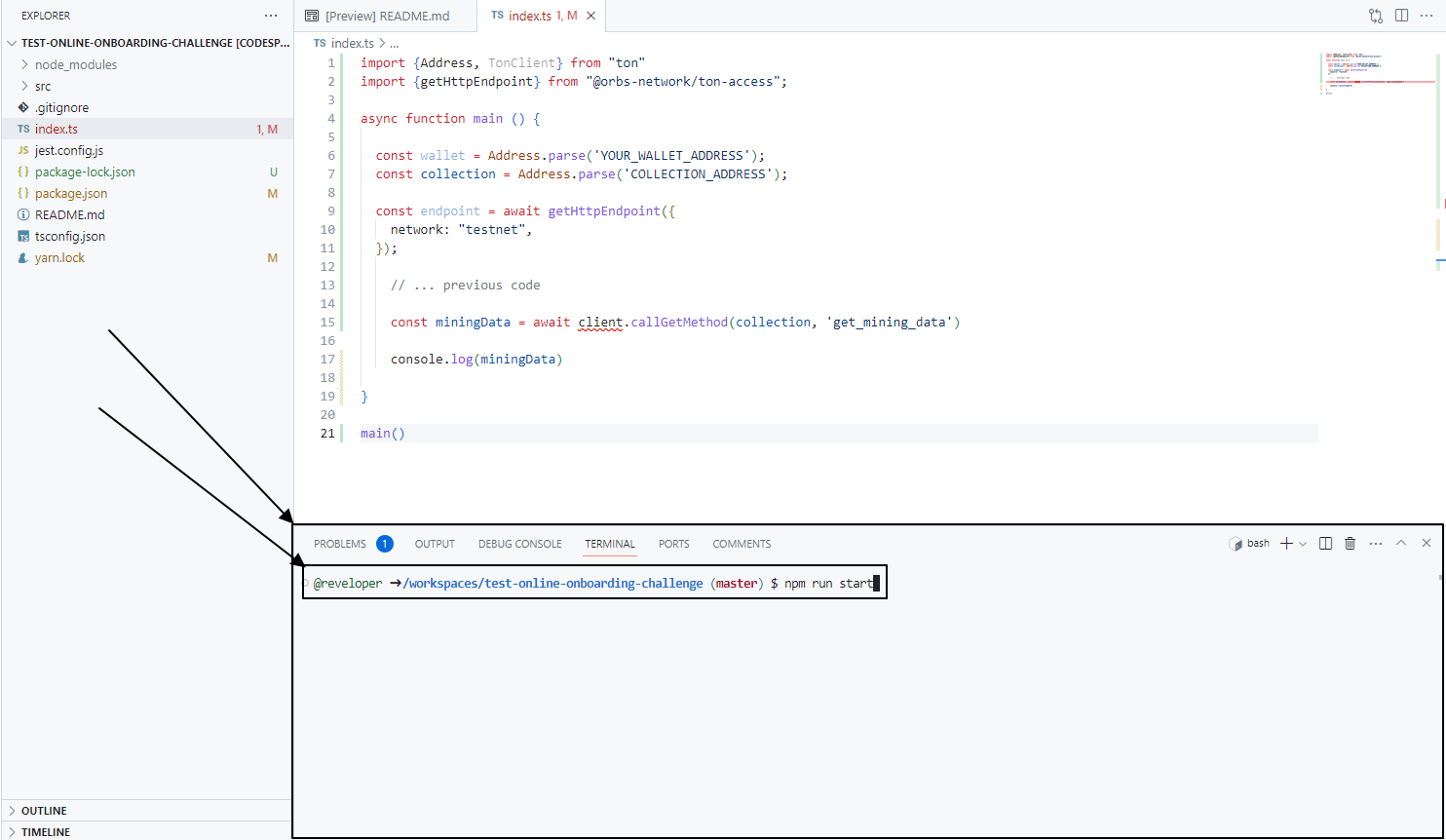
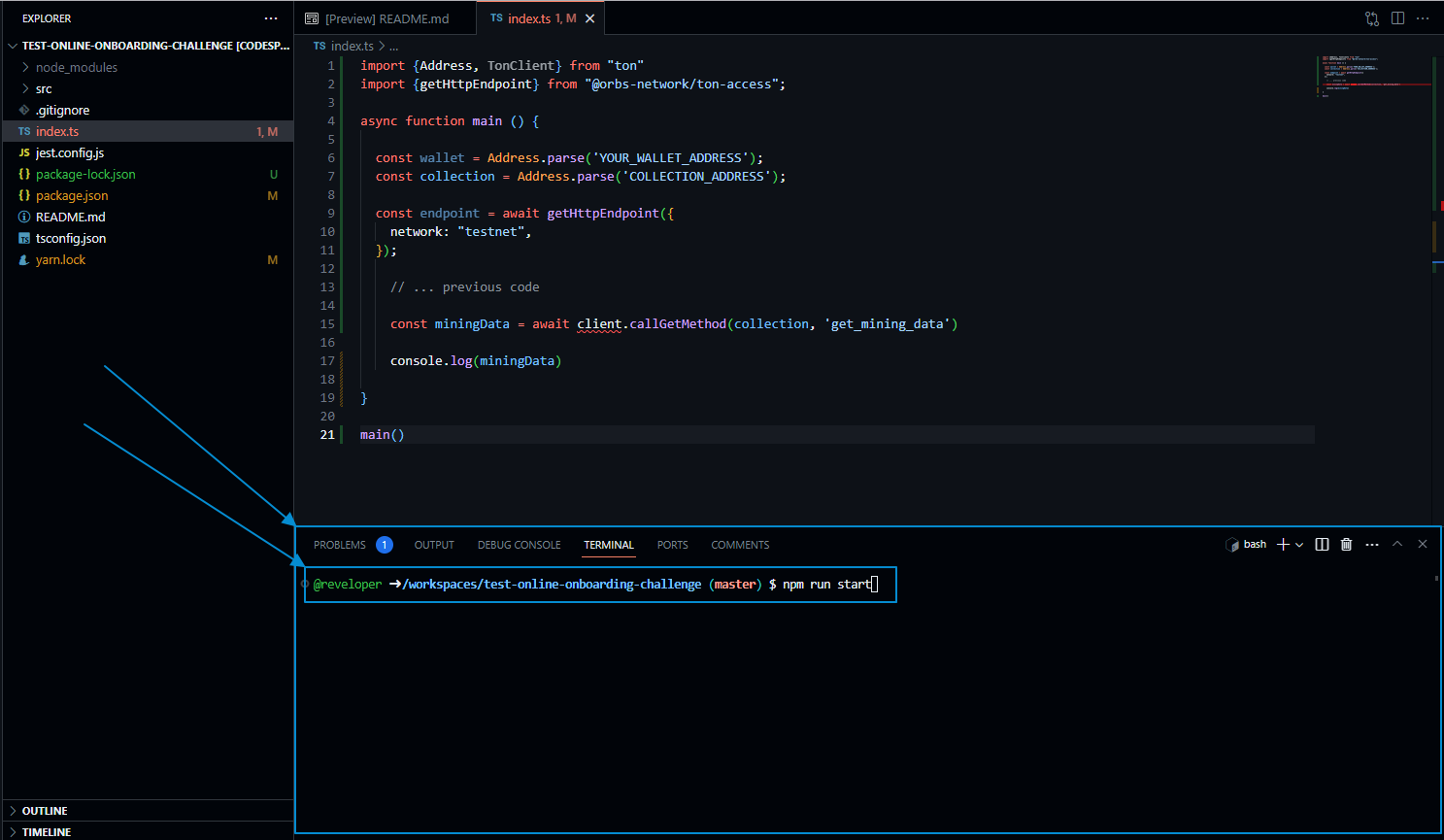
终端也可作为单独的应用程序使用。请根据您的集成开发环境和操作系统选择合适的版本。
太好了!完成这些步骤后,您就可以深入了解 TON 区块链的秘密了。👀
🎯 连接到 TON
好了,连接 TON 区块链需要什么?
- 智能合约地址 作为目的地。我们的目标是从 工作防伪智能合约 中挖掘一个 NFT,因此我们需要一个地址来获取当前的挖矿复杂度。
- API provider 向 TON 区块链提出请求。TON 有多种 API 类型,用于不同目的。我们将使用 toncenter.com API 的 testnet 版本。
- JavaScript SDK:需要使用 JavaScript SDK(请注意,SDK 是软件开发工具包)来解析正在使用的智能合约地址,并为创建 API 请求做好准备。为了更好地理解 TON 地址以及为什么需要对其进行解析以执行此过程,请参阅此 资源,以了解我们为什么要对其进行解析。为了执行此过程,我们将使用
@ton/ton
。
下一节我们将介绍用户如何使用 TONCenter API 和@ton/ton
向TON区块链发送初始请求,以便从PoW智能合约接收数据。
智能合约地址
为了让矿工正常工作,我们需要添加两种不同的智能合约地址类型。其中包括